Memory Leak in .NET
Problem
Your program stops due to memory leak when using C#. You can check this by opening Task Manager → Performance and see how much of GPU memory is used while running your program.
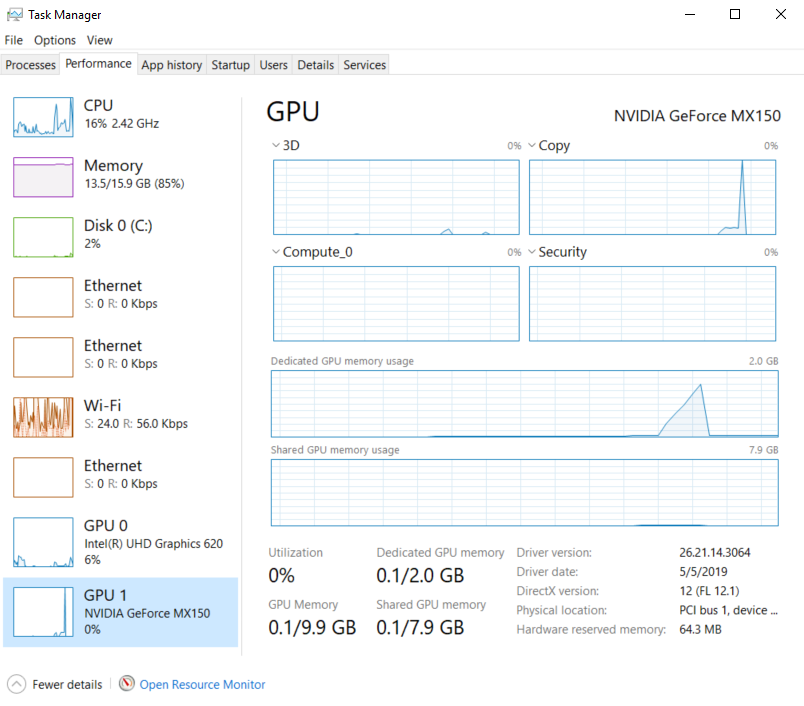
Possible cause
The memory leak often occurs because your IDisposable objects have not been disposed of. The garbage collector handles application memory and makes sure to clean up unreferenced objects before running out of memory. However, the garbage collector is not aware of GPU memory usage. Therefore it may not clean up in time before GPU memory fills up.
The example code below causes a memory leak when capturing in a loop an HDR image consisting of 3 acquisitions.
// This code results in memory leak.
using System;
using System.Collections.Generic;
class memoryLeak
{
static int Main()
{
try
{
var zivid = new Zivid.NET.Application();
Console.WriteLine("Connecting to camera");
var camera = zivid.ConnectCamera();
Console.WriteLine("Configuring settings");
var settings = new Zivid.NET.Settings();
foreach (var aperture in new double[] { 9.57, 4.76, 2.59 })
{
Console.WriteLine("Adding acquisition with aperture = " + aperture);
var acquisitionSettings = new Zivid.NET.Settings.Acquisition { Aperture = aperture };
settings.Acquisitions.Add(acquisitionSettings);
}
while (true)
{
var frame = camera.Capture3D(settings);
Console.WriteLine("Captured frame (HDR)");
}
}
catch (Exception ex)
{
Console.WriteLine("Error: " + ex.Message);
return 1;
}
return 0;
}
}
What happens is that var frame = camera.Capture3D(settings)
creates a new object frame
.
This object is not disposed when capturing the next frame.
Solution
To mitigate this it is recommended to force deterministic memory cleanup by using the using
keyword.
using (var frame = camera.Capture3D(settings))
{
Console.WriteLine("Captured frame (HDR)");
}
It is also possible to explicit dispose the frame after each capture.
var frame = camera.Capture3D(settings);
Console.WriteLine("Captured frame (HDR)");
frame.Dispose();
You can confirm that you no longer have a memory leak by checking the memory usage in task manager.
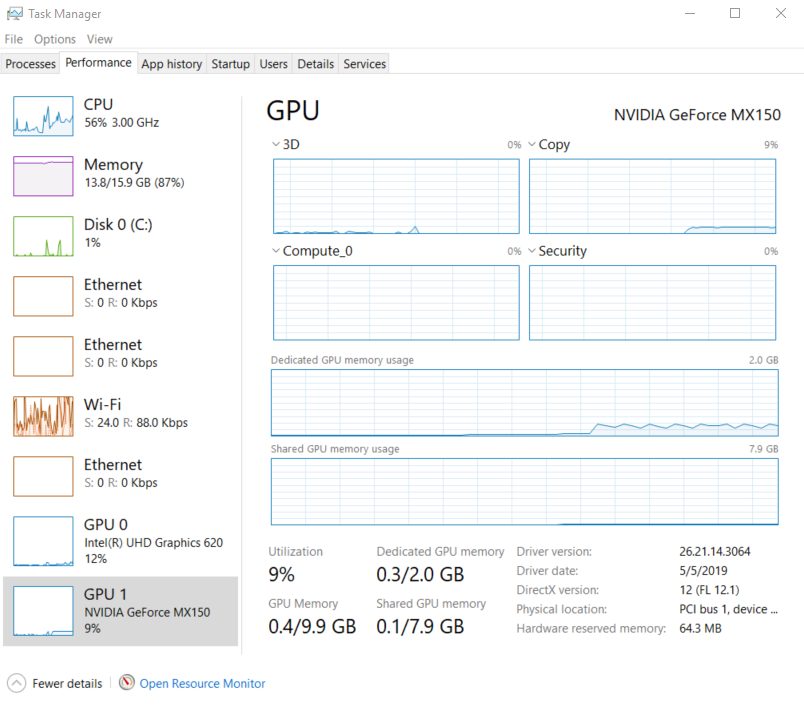